ELECTRONICS AND CONTROL
Electronic board
The brain of the project is in a single electronic board, I used a modification of my Atmega used in week 16 and whose first design was in week 9.
I analyzed the quantities of inputs and outputs needed to place the terminals for a more solid wiring. The design used is as follows:
And here is the Board.
Finally, my Vaporex Board is ready!
As you can see, apart from the sensors I used some LEDs that will indicate its operation and an LCD screen of the 20x4 format using the I2C communication protocol(Output week).
By using this communication protocol(week14), I get less wiring with my electronic board.
Thanks to this screen I will be able to visualize all the measured variables: temperature, pressure and level.
Control logic/Programming (Embedded programming)
When the machine is activated the microcontroller will make a measurement of all the values of the sensors and will show a message indicating that the system is ready.
In our project it will work under 4 main conditions that will allow the operation or not of our machine:
- The deposit must be above the level of resistance. Failure to comply with this condition could cause deterioration in a short period of time.
- The pressure must not exceed 3BAR, to avoid an overload of pressure and can explode as if it were a small bomb
- The interior temperature should not exceed 95º, if it exceeds this temperature the pressure will begin to increase, and we may have a problem.
- If the water level is above the maximum, you can enter our pressure sensor and cause a breakdown
When the resistance is activated and the temperature value exceeds 23º, the pressure is less than 1 and the temperature is below 70º, a message of "wait, heat up" will be displayed and a white LED will be activated.
When it exceeds 70º and is below 3BAR, a green LED will be activated and a signal that says: "You can use the steam".
In the event that the temperature exceeds 90º, is below 95 and the pressure is below 3bar, the relay signal will be deactivated by disconnecting the resistance, but the steam message will follow and the green led will remain.
In the event that it exceeds 100º or the pressure exceeds 3BAR, the resistance will be immediately deactivated, an alert message will be output and the red led will activate.
Next, a picture of its operation.
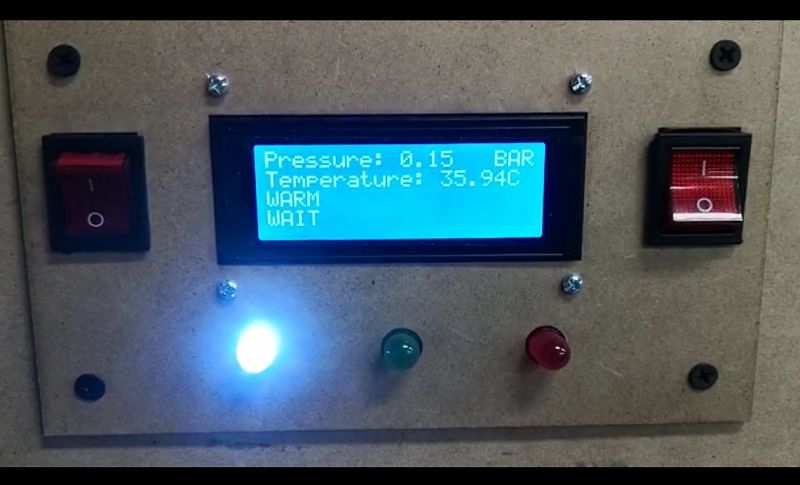
Code
Here is a final code:
#include "Wire.h>
#include "LiquidCrystal_I2C.h>
#include "SoftwareSerial.h>
#include "OneWire.h>
#include "DallasTemperature.h>
SoftwareSerial mySerial(0,1);
LiquidCrystal_I2C lcd(0x27,20,4); // set the LCD address to 0x27 for a 16 chars and 2 line display
#define ONE_WIRE_BUS 16
OneWire oneWire(ONE_WIRE_BUS);
DallasTemperature sensors(&oneWire);
const float offset=1.0;
int rele=11;
int pinNivel1 = 8;//BAJO
int pinNivel2 = 9;//MITAD
int pinNivel3 = 10;//LLENO
int estadoNivelAgua1 = 0;//BAJO
int estadoNivelAgua2 = 0;//MEDIO
int estadoNivelAgua3 = 0;//LLENO
int rojo=6;
int blanco=5;
int verde=7;
void setup()
{
sensors.begin();
pinMode(rele,OUTPUT);
// pinMode(pinNivel1, INPUT);
// pinMode(pinNivel2, INPUT);
// pinMode(pinNivel3, INPUT);
pinMode(rojo,OUTPUT);
pinMode(blanco,OUTPUT);
pinMode(verde,OUTPUT);
digitalWrite(rele,HIGH);
lcd.init(); // initialize the lcd
lcd.init();
// Print a message to the LCD.
lcd.backlight();
lcd.setCursor(7,1);
lcd.print("Vaporex");
delay(2500);
lcd.setCursor(7,1);
lcd.print(" ");
lcd.setCursor(0,0);
lcd.print("Data adquisition");
float sensorValue = (analogRead(A3)*7.0/1023.0)-offset;
lcd.setCursor(0,1);
lcd.print("Pressure: ");
lcd.setCursor(0,2);
lcd.print("Temperature: ");
lcd.setCursor(13,1);
lcd.print(sensorValue);
sensors.requestTemperatures();
lcd.setCursor(13,2);
lcd.print(sensors.getTempCByIndex(0));
delay(2500);
lcd.clear();
lcd.setCursor(7,1);
lcd.print("System");
lcd.setCursor(7,2);
lcd.print("Ready");
delay(2500);
mySerial.begin(9600);
}
void loop()
{
float sensorValue = (analogRead(A3)*7.0/1023.0)-offset;
sensors.requestTemperatures();
float temp=sensors.getTempCByIndex(0);
estadoNivelAgua1 = digitalRead(pinNivel1);
estadoNivelAgua2 = digitalRead(pinNivel2);
estadoNivelAgua3 = digitalRead(pinNivel3);
if (estadoNivelAgua1 == HIGH && estadoNivelAgua2 == HIGH && estadoNivelAgua3 == HIGH) {
mySerial.println("Full level");
digitalWrite(rojo,LOW);
digitalWrite(blanco,LOW);
digitalWrite(verde,HIGH);
}
if (estadoNivelAgua1 == LOW && estadoNivelAgua2 == HIGH && estadoNivelAgua3 == HIGH) {
mySerial.println("Medium level");
digitalWrite(rojo,LOW);
digitalWrite(blanco,HIGH);
digitalWrite(verde,LOW);
}
if (estadoNivelAgua1 == LOW && estadoNivelAgua2 == LOW && estadoNivelAgua3 == HIGH ) {
mySerial.println("low water level");
digitalWrite(rojo,HIGH);
digitalWrite(blanco,LOW);
digitalWrite(verde,LOW);
}
if(estadoNivelAgua1 == LOW && estadoNivelAgua2 == LOW && estadoNivelAgua3 == LOW){
mySerial.println("Tank empty.");
digitalWrite(rojo,HIGH);
digitalWrite(blanco,LOW);
digitalWrite(verde,LOW);
}
lcd.clear();
lcd.setCursor(0,0);
lcd.print("Pressure: ");
lcd.setCursor(0,1);
lcd.print("Temperature: ");
lcd.setCursor(10,0);
lcd.print(" ");
lcd.setCursor(10,0);
lcd.print(sensorValue);
lcd.setCursor(17,0);
lcd.print("BAR");
lcd.setCursor(13,1);
lcd.print(temp);
lcd.setCursor(18,1);
lcd.print("C");
mySerial.println(sensorValue);
if(temp(<)22.0 && sensorValue(<)1.0){
lcd.setCursor(0,2);
lcd.print("LOW TTEMPERATURE");
lcd.setCursor(0,3);
lcd.print("JUST IRONING");
digitalWrite(rojo,HIGH);
digitalWrite(blanco,LOW);
digitalWrite(verde,LOW);
digitalWrite(rele,LOW);
}
if(temp(>)22.0 && temp(<)89.0 && sensorValue(<)3.0){
digitalWrite(rele,LOW);
digitalWrite(blanco,HIGH);
digitalWrite(rojo,LOW);
digitalWrite(verde,LOW);
lcd.setCursor(0,2);
lcd.print("WARM");
lcd.setCursor(0,3);
lcd.print("WAIT");
}
if(temp(>)90.0 && sensorValue(<)3.0){
digitalWrite(rele,LOW);
digitalWrite(verde,HIGH);
digitalWrite(blanco,LOW);
digitalWrite(rojo,LOW);
lcd.setCursor(0,2);
lcd.print("TEMPERATURE OK");
lcd.setCursor(0,3);
lcd.print("STEAM READY");
}
if(temp(>)95.0 || sensorValue(>=)3.0){
digitalWrite(rele,HIGH);
digitalWrite(rojo,LOW);
digitalWrite(blanco,LOW);
digitalWrite(verde,HIGH);
}
if(temp(>)100.0 || sensorValue(>=)3.0 || estadoNivelAgua1 == LOW && estadoNivelAgua2 == LOW && estadoNivelAgua3 == LOW || estadoNivelAgua1 == LOW && estadoNivelAgua2 == HIGH && estadoNivelAgua3 == HIGH){
digitalWrite(rele,HIGH);
digitalWrite(rojo,HIGH);
digitalWrite(blanco,LOW);
digitalWrite(verde,LOW);
lcd.setCursor(0,2);
lcd.print("WARNING");
lcd.setCursor(0,3);
lcd.print("WAIT");
}
delay(500);
}
Return to final project
Download
You can download this files Here:
Code
Final code
Board
Vaporex traces
Vaporex out traces
Vaporex schematic
Vaporex brd